4. Creating the <Product>
Component¶
Note
A best practise in designing web application front ends, and especially when utilizing the ReactJS library, is to breakdown the final design into modular, portable and reusable components.
Take a second and think about the components that you could break this up into. Remembering that thus far we have defined the <ProductRegistry>
.
- Can the interface be simplified to a
<ProductRegistry>
of<Products>
? We think so!
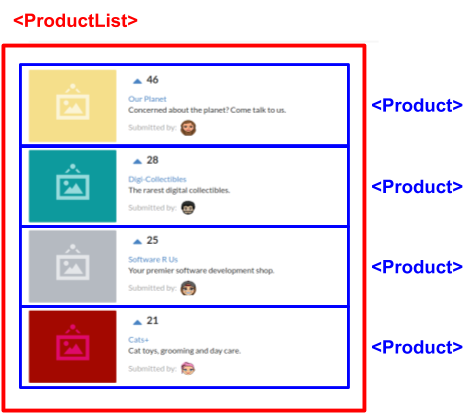
Navigate back to your open
app.js
file in your text editor. It should currently look like this:class ProductRegistry extends React.Component { render() { return ( <div className='ui unstackable items'> Hello, I am your first React component! </div> ); } } ReactDOM.render( <ProductRegistry />, document.getElementById('content') );
Begin by defining a brand new component, JavaScript class, beneath the existing
<ProductRegistry>
componentclass Product extends React.Component {}
This is a completely empty component that will not render anything and in fact will throw an error as a
render()
function is required for each component. This is the function that defines what is to be rendered by the browser and by default empty components are not allowed.Add a
render()
function to the<Product>
component to return a simple<div>
saying “hello”class Product extends React.Component { render() { return ( <div>Hello I am a product.</div> ); } }
Now remember what is currently being rendered to the page:
ReactDOM.render( <ProductRegistry />, document.getElementById('content') );
Therefore the
<Product>
component is not being rendered yet and will not be present in the browserAdd the
<Product>
component to the components that are returned by your<ProductRegistry>
within theapp.js
fileclass ProductRegistry extends React.Component { render() { return ( <div className='ui unstackable items'> Hello, I am your first React component! <Product /> </div> ); } }
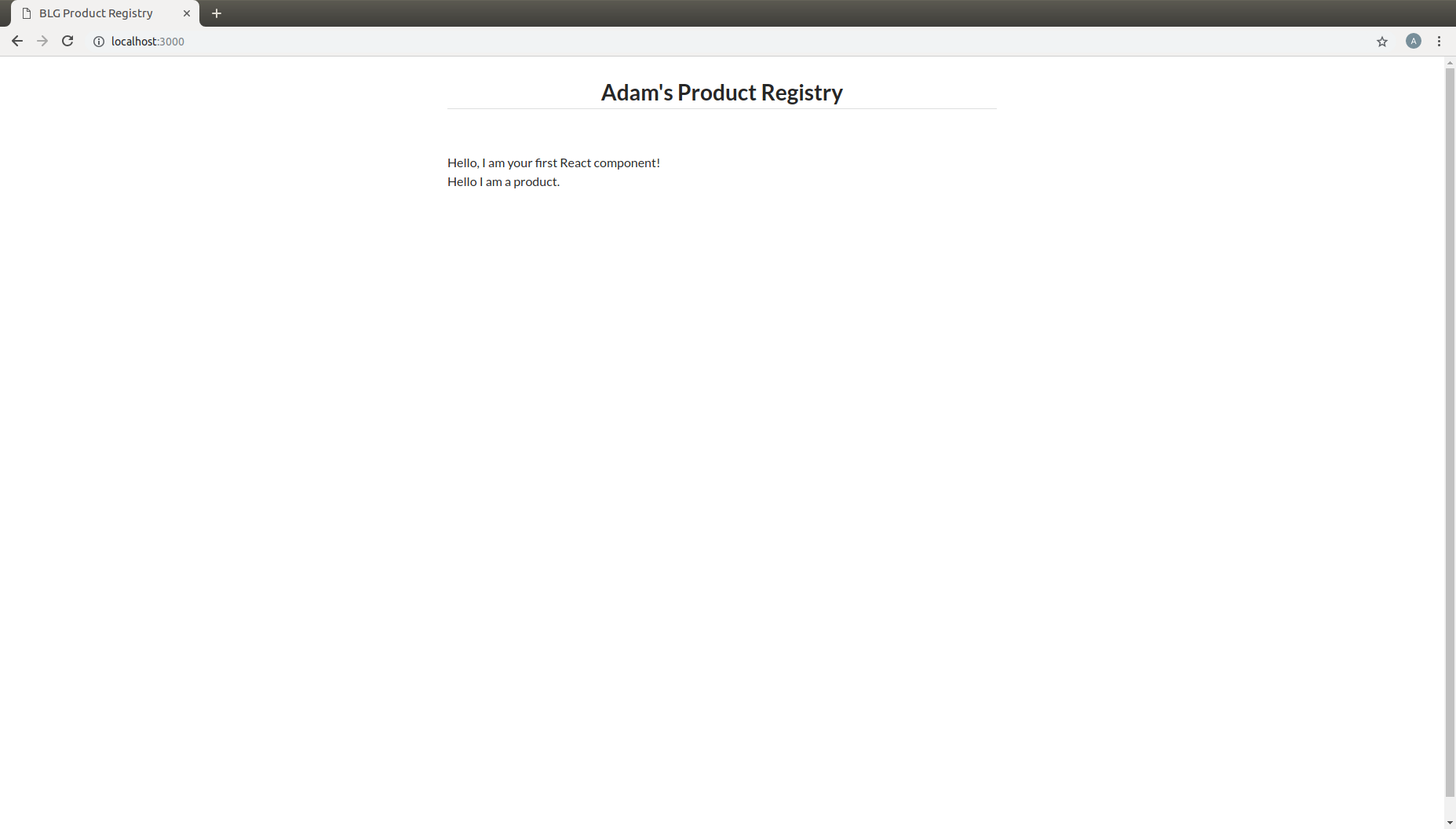